Using Authsignal with Auth0
Learn how to integrate Authsignal with Auth0 to add MFA to your login flow, using methods such as Authenticator App, SMS OTP, Email OTP, and Passkeys.
This guide will demonstrate how to integrate Authsignal with Auth0 by creating a Custom Action for the Login / Post-Login trigger.
The end result will be to allow users to complete an MFA challenge after they login with their username and password, redirecting them from the Auth0 Universal Login Page to Authsignal’s pre-built UI.
The integration follows Auth0’s official guidelines for redirecting users after login to do custom MFA. You can learn more here about how to Redirect with Actions in Auth0.
User flow
The user enters their username and password in the Auth0 Universal Login page
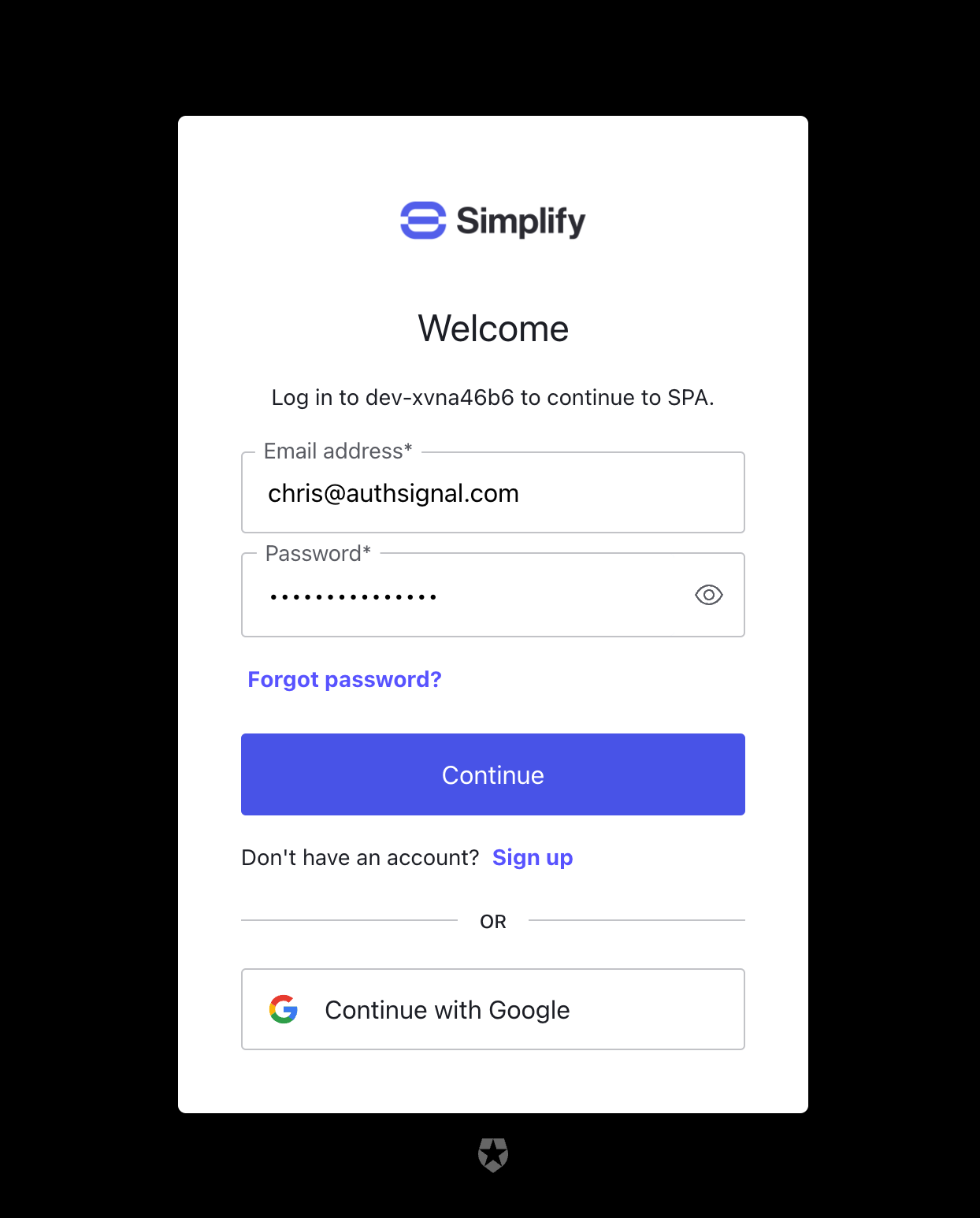
The user is redirected to Authsignal's pre-built UI to complete an MFA challenge
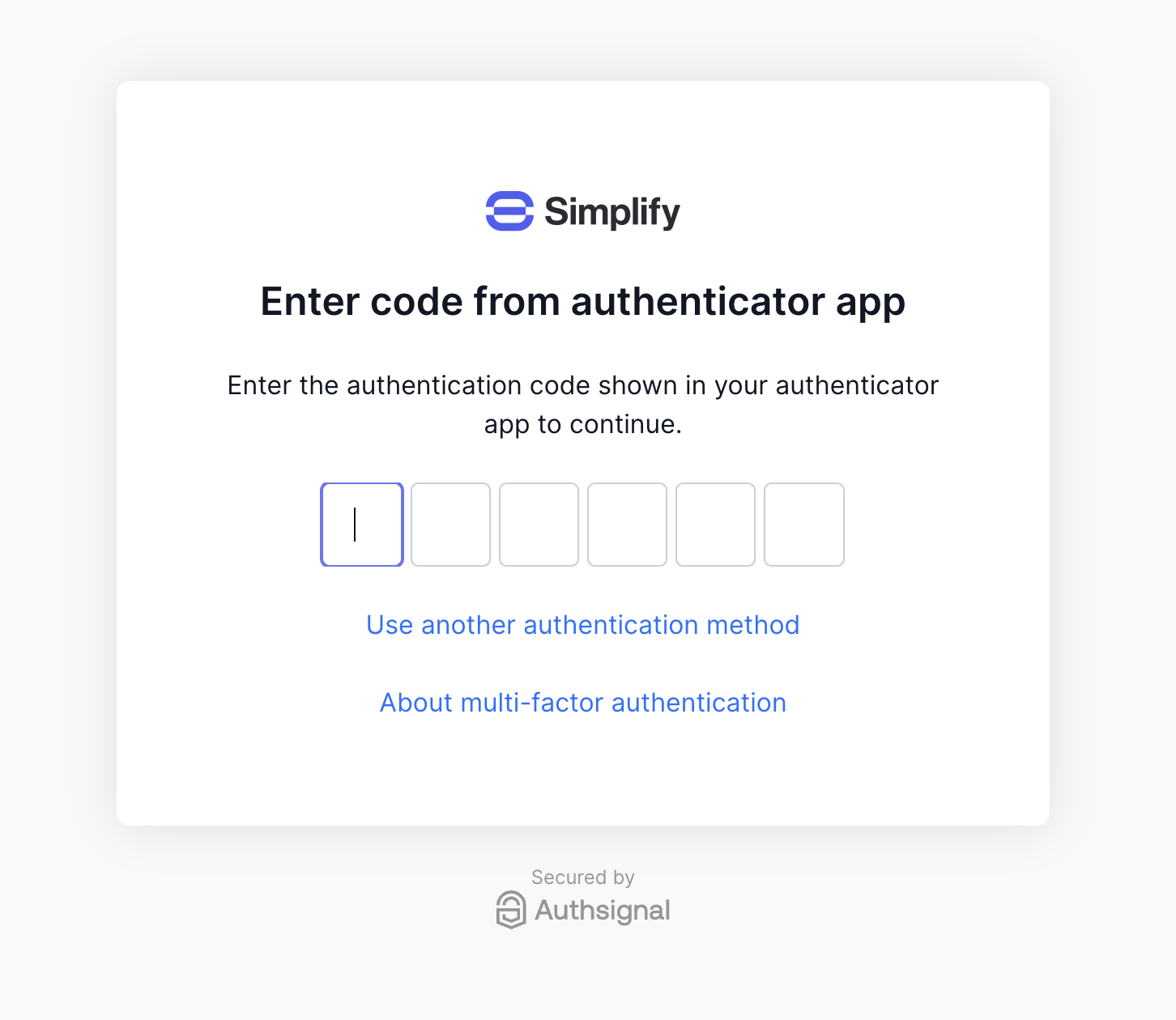
The user is logged in if the challenge is completed successfully
Integration steps
Create an action
In the Auth0 Dashboard, go to Actions → Library and select “Build Custom”. Then select the “Login / Post Login” trigger and give the action an appropriate name (for example “post-login-mfa”).
Add your secret
Now your action has been created, create a new secret called AUTHSIGNAL_SECRET
and provide the value of your secret from the Api Keys section in the Authsignal Portal.
Add dependencies
Add the @authsignal/node
dependency.
Add the action code
Add the following code snippet to the action.
const { handleAuth0ExecutePostLogin, handleAuth0ContinuePostLogin } = require("@authsignal/node");
exports.onExecutePostLogin = handleAuth0ExecutePostLogin;
exports.onContinuePostLogin = handleAuth0ContinuePostLogin;
If using a non-US region (e.g. AU or EU) you will need to override the base URL as follows:
const { handleAuth0ExecutePostLogin, handleAuth0ContinuePostLogin } = require("@authsignal/node");
const apiBaseUrl = "https://eu.api.authsignal.com/v1";
exports.onExecutePostLogin = async (event, api) => {
await handleAuth0ExecutePostLogin(event, api, { apiBaseUrl });
};
exports.onContinuePostLogin = async (event, api) => {
await handleAuth0ContinuePostLogin(event, api, { apiBaseUrl });
};
Connect your action to the Login flow
Now connect your action in the Flows section of the Auth0 Dashboard by dragging it into the Login flow.
Configure the action in Authsignal
Once the previous steps have been completed, the next time the Auth0 action is run you will see an action appear in the Authsignal Portal called “Auth0 Login”. You will need to set the default outcome for this action to Challenge
and save it.
That’s it! You’ve done everything required to add MFA to your Auth0 login flow using Authsignal.
Next steps
Was this page helpful?